Modify the HTML in last post
Add custom control on Google Map with "viewport" added, to make it suitable for mobile device.
The HTML displayed on PC, Google Chrome.

The HTML displayed on Android, Mobile Opera.
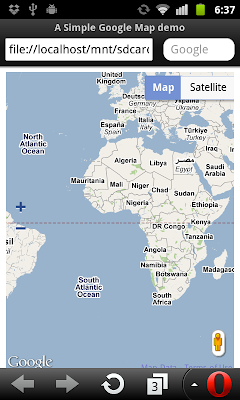
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="initial-scale=1.0, user-scalable=no"/>
<title>A Simple Google Map demo</title>
<style type="text/css">
html { height: 100% }
body { height: 100%}
#mymap { height: 100% }
</style>
<script type="text/javascript" src="http://maps.google.com/maps/api/js?sensor=false"></script>
<script type="text/javascript">
var map;
function InitMap(){
var options = {
zoom: 2,
center: new google.maps.LatLng(0, 0),
mapTypeId: google.maps.MapTypeId.ROADMAP,
zoomControl: true,
zoomControlOptions: {
style: google.maps.ZoomControlStyle.LARGE,
position: google.maps.ControlPosition.LEFT_CENTER},
streetViewControl: true,
streetViewControlOptions: {
position: google.maps.ControlPosition.RIGHT_BOTTOM}
};
map = new google.maps.Map(document.getElementById("mymap"), options);
}
function htmlonload(){
InitMap();
}
</script>
</head>
<body onload="htmlonload();">
<div id="mymap"></div>
</body>
</html>
Next:
-
Tracking my location on mobile web with Google Map