To enable the code assist for jQuery in Aptana Studio 3, Download and Add the .sdocml file here: https://github.com/aptana/javascript-jquery.ruble/tree/master/support
Thursday, October 27, 2011
Add code assist for jQuery in Aptana
Labels:
Development Tools,
how to,
jQuery
Friday, October 21, 2011
Implement Slider using HTML5 an JavaScript
This example show how to implement a slider using HTML5 and JavaScript. To demonstrate the effect, code from "jQuery exercise: change font-size" is borrowed, to change text size when slide is changed.
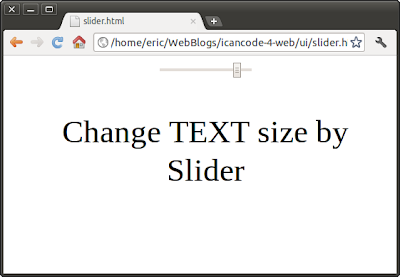
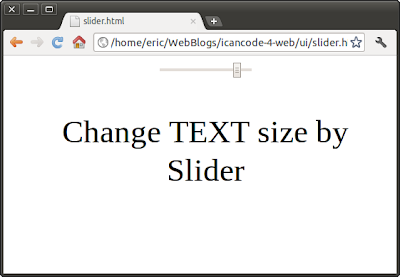
<!DOCTYPE html>
<html>
<head>
<script src="http://code.jquery.com/jquery-latest.js"></script>
<style>
body { margin-left: 30px; margin-right: 15px; background-color: #ffffff }
h1{font: bold italic 20pt helvetica}
#mytext {
font-size: 20px
}
</style>
</head>
<body>
<center>
<input id="slide" type="range" min="8" max="50" value="20"? onChange="changeTextSize(this.value)" step="1"/>
<p id="mytext">Change TEXT size by Slider</p>
</center>
<script>
function changeTextSize(fontsize) {
$("#mytext").css("font-size", fontsize+"px");
}
</script>
</body>
</html>
Labels:
HTML5,
JavaScript Exercises
Thursday, October 20, 2011
Javascript Exercise: Get text and change text
example:


<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Get input</title>
</head>
<body>
<h1>Get input and change text</h1>
<p id="mytextchanged">Hello!</p>
<input id="mytext" type="text">
<input type="button" value="Click Me" onClick="showMe()" />
<script type="text/javascript">
function showMe() {
var msg = document.getElementById("mytext").value;
document.getElementById("mytextchanged").innerHTML = msg;
}
</script>
</body>
</html>
Labels:
JavaScript Exercises
Read input text using JavaScript
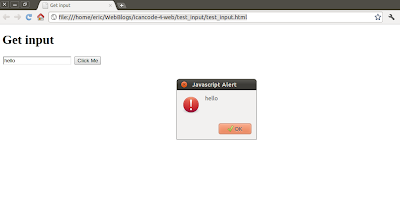
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Get input</title>
</head>
<body>
<h1>Get input</h1>
<input id="mytext" type="text">
<input type="button" value="Click Me" onClick="showMe()" />
<script type="text/javascript">
function showMe() {
var msg = document.getElementById("mytext").value;
alert(msg);
}
</script>
</body>
</html>
Labels:
JavaScript Exercises
Monday, October 17, 2011
Play mp4 using HTML5
Example to play mp4 in HTML5.

<!DOCTYPE html>
<html>
<head>
</head>
<body>
<video src="http://video.ch9.ms/ch9/51fe/77b82ce9-bbbc-4b9c-b19a-9f6b017551fe/MVP1understandingwptools_low_ch9.mp4"
controls autoplay loop>
<p>Sorry! Your browser doesn't support video.</p>
</video>
</body>
</html>

Labels:
HTML5
Friday, October 14, 2011
jQuery exercise: change font-size

<!DOCTYPE html>
<html>
<head>
<script src="http://code.jquery.com/jquery-latest.js"></script>
<style>
body { margin-left: 30px; margin-right: 15px; background-color: #ffffff }
h1{font: bold italic 20pt helvetica}
#mytext {
font-size: 20px
}
</style>
</head>
<body>
<center>
<p id="mytext">Change TEXT size by UP/DOWN key</p>
</center>
<script>
$(function(){
var KEY_UP = 38;
var KEY_DOWN = 40;
//Set listener for keydown
$(document).keydown(function(e){
$("#mytext2").html("- keydown -");
switch(e.which){
case KEY_UP:
var fontsize = parseInt($("#mytext").css("font-size")) + 2;
$("#mytext").css("font-size", fontsize+"px");
break;
case KEY_DOWN:
var fontsize = parseInt($("#mytext").css("font-size")) - 2;
$("#mytext").css("font-size", fontsize+"px");
break;
}
});
});
</script>
</body>
</html>
Related: Implement Slider using HTML5 an JavaScript
Labels:
CSS,
JavaScript,
jQuery
Thursday, October 13, 2011
jQuery exercise: get key input, to change CSS layout.
By merging of the exercises "jQuery exercise: get key input" and "Apply style on div", we can change the red dot position using JavaScript. When user press (also press and hold) UP, DOWN, LEFT or RIGHT key can move the red dot position.
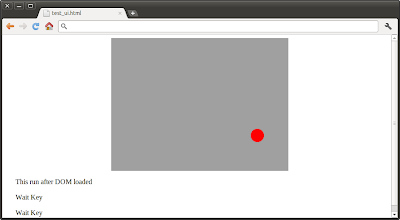
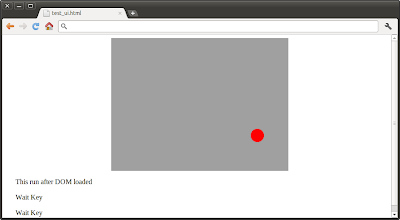
<!DOCTYPE html>
<html>
<head>
<script src="http://code.jquery.com/jquery-latest.js"></script>
<style>
body { margin-left: 30px; margin-right: 15px; background-color: #ffffff }
h1{font: bold italic 20pt helvetica}
#background{
background: #a0a0a0;
width: 400px;
height: 300px;
position: relative;
overflow: hidden;
}
#reddot {
background: #f00;
position: absolute;
width: 30px;
height: 30px;
left: 185px;
top: 135px;
border-radius: 15px;
}
</style>
</head>
<body>
<center>
<div id="background">
<div id="reddot"></div>
</div>
</center>
<p id="mytext1">Original Text</p>
<p id="mytext2">Wait Key</p>
<p id="mytext3">Wait Key</p>
<script>
$(function(){
var str = "This run after DOM loaded";
$("#mytext1").html(str);
var KEY_UP = 38;
var KEY_DOWN = 40;
var KEY_LEFT = 37;
var KEY_RIGHT = 39;
//Set listener for keydown
$(document).keydown(function(e){
$("#mytext2").html("- keydown -");
switch(e.which){
case KEY_UP:
var top = parseInt($("#reddot").css("top")) - 10;
$("#reddot").css("top", top);
$("#mytext3").html("Key: UP / " + left + " : " + top);
break;
case KEY_DOWN:
var top = parseInt($("#reddot").css("top")) + 10;
$("#reddot").css("top", top);
$("#mytext3").html("Key: DOWN / " + left + " : " + top);
break;
case KEY_LEFT:
var left = parseInt($("#reddot").css("left")) - 10;
$("#reddot").css("left", left);
$("#mytext3").html("Key: LEFT / " + left + " : " + top);
break;
case KEY_RIGHT:
var left = parseInt($("#reddot").css("left")) + 10;
$("#reddot").css("left", left);
$("#mytext3").html("Key: RIGHT / " + left + " : " + top);
break;
}
});
//Set listener for keyup
$(document).keyup(function(e){
$("#mytext2").html("Wait Key");
$("#mytext3").html("Wait Key");
});
});
</script>
</body>
</html>
Labels:
CSS,
JavaScript,
jQuery
Convert CSS width or height to int using JavaScript
Normal, width or height defined in CSS as "px", "cm". It can be converted to int using JavaScript function parseInt(string, radix).
The parseInt function produces an integer value dictated by interpretation of the contents of the string argument according to the specified radix. Leading white space in string is ignored. If radix is undefined or 0, it is assumed to be 10 except when the number begins with the character pairs 0x or 0X, in which case a radix of 16 is assumed. If radix is 16, the number may also optionally begin with the character pairs 0x or 0X.
Example: jQuery exercise: get key input, to change CSS layout.
The parseInt function produces an integer value dictated by interpretation of the contents of the string argument according to the specified radix. Leading white space in string is ignored. If radix is undefined or 0, it is assumed to be 10 except when the number begins with the character pairs 0x or 0X, in which case a radix of 16 is assumed. If radix is 16, the number may also optionally begin with the character pairs 0x or 0X.
Example: jQuery exercise: get key input, to change CSS layout.
Labels:
CSS,
JavaScript
Wednesday, October 12, 2011
jQuery exercise: get key input
<!DOCTYPE html>
<html>
<head>
<script src="http://code.jquery.com/jquery-latest.js"></script>
</head>
<body>
<p id="mytext1">Original Text</p>
<p id="mytext2">Wait Key</p>
<p id="mytext3">Wait Key</p>
<script>
$(function(){
var str = "This run after DOM loaded";
$("#mytext1").html(str);
var KEY_UP = 38;
var KEY_DOWN = 40;
var KEY_LEFT = 37;
var KEY_RIGHT = 39;
//Set listener for keydown
$(document).keydown(function(e){
$("#mytext2").html("- keydown -");
switch(e.which){
case KEY_UP:
$("#mytext3").html("Key: UP");
break;
case KEY_DOWN:
$("#mytext3").html("Key: DOWN");
break;
case KEY_LEFT:
$("#mytext3").html("Key: LEFT");
break;
case KEY_RIGHT:
$("#mytext3").html("Key: RIGHT");
break;
}
});
//Set listener for keyup
$(document).keyup(function(e){
$("#mytext2").html("Wait Key");
$("#mytext3").html("Wait Key");
});
});
</script>
</body>
</html>

Labels:
jQuery
jQuery exercise: change text
Exercise to change text using jQuery.
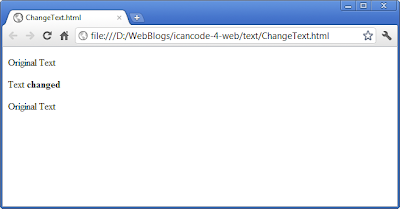
<!DOCTYPE html>
<html>
<head>
<script src="http://code.jquery.com/jquery-latest.js"></script>
</head>
<body>
<p id="mytext1">Original Text</p>
<p id="mytext2">Original Text</p>
<p id="mytext3">Original Text</p>
<script>
var str = "Text <b>changed</b>";
$("#mytext2").html(str);
</script>
</body>
</html>
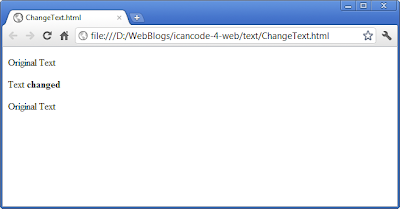
Labels:
jQuery
Saturday, October 1, 2011
Load Feed with Google Feed API
With the Feed API, you can download any public Atom, RSS, or Media RSS feed using only JavaScript.
The Google Feed API takes the pain out of developing mashups in JavaScript because you can now mash up feeds using only a few lines of JavaScript, rather than dealing with complex server-side proxies. This makes it easy to quickly integrate feeds on your website.
Know more: Google Feed API
Example:

The Google Feed API takes the pain out of developing mashups in JavaScript because you can now mash up feeds using only a few lines of JavaScript, rather than dealing with complex server-side proxies. This makes it easy to quickly integrate feeds on your website.
Know more: Google Feed API
Example:
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="content-type" content="text/html; charset=utf-8"/>
<title>Google AJAX Search API Sample</title>
<script src="http://www.google.com/jsapi?key=AIzaSyA5m1Nc8ws2BbmPRwKu5gFradvD_hgq6G0" type="text/javascript"></script>
<script type="text/javascript">
/*
* How to load a feed via the Feeds API.
*/
google.load("feeds", "1");
// Our callback function, for when a feed is loaded.
function feedLoaded(result) {
if (!result.error) {
// Grab the container we will put the results into
var container = document.getElementById("content");
container.innerHTML = '';
// Loop through the feeds, putting the titles onto the page.
// Check out the result object for a list of properties returned in each entry.
// http://code.google.com/apis/ajaxfeeds/documentation/reference.html#JSON
for (var i = 0; i < result.feed.entries.length; i++) {
var entry = result.feed.entries[i];
var div = document.createElement("div");
div.appendChild(document.createTextNode(entry.title));
container.appendChild(div);
}
}
}
function OnLoad() {
// Create a feed instance that will grab Digg's feed.
var feed = new google.feeds.Feed("http://feeds.feedburner.com/ICanCodeForWeb");
// Calling load sends the request off. It requires a callback function.
feed.load(feedLoaded);
}
google.setOnLoadCallback(OnLoad);
</script>
</head>
<body style="font-family: Arial;border: 0 none;">
<div id="content">Loading...</div>
</body>
</html>

Labels:
Google APIs
Subscribe to:
Posts (Atom)