example:
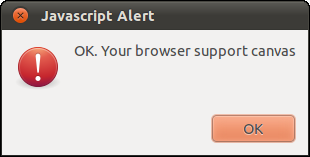
<!DOCTYPE html>
<html>
<head>
<title>JavaScript Exercise</title>
<script type="text/javascript">
function CheckCanvas(){
if(document.createElement('canvas').getContext){
alert("OK. Your browser support canvas");
}else{
alert("Sorry! your brwser doesn't support canvas");
}
}
function htmlonload(){
CheckCanvas();
}
</script>
</head>
<body onload="htmlonload();">
JavaScript Exercise
</body>
</html>