By merging of the exercises "
jQuery exercise: get key input" and "
Apply style on div", we can change the red dot position using JavaScript. When user press (also press and hold) UP, DOWN, LEFT or RIGHT key can move the red dot position.
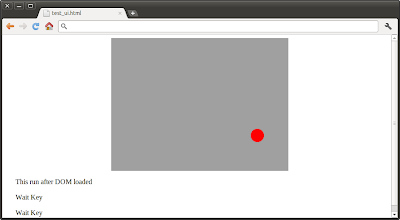
<!DOCTYPE html>
<html>
<head>
<script src="http://code.jquery.com/jquery-latest.js"></script>
<style>
body { margin-left: 30px; margin-right: 15px; background-color: #ffffff }
h1{font: bold italic 20pt helvetica}
#background{
background: #a0a0a0;
width: 400px;
height: 300px;
position: relative;
overflow: hidden;
}
#reddot {
background: #f00;
position: absolute;
width: 30px;
height: 30px;
left: 185px;
top: 135px;
border-radius: 15px;
}
</style>
</head>
<body>
<center>
<div id="background">
<div id="reddot"></div>
</div>
</center>
<p id="mytext1">Original Text</p>
<p id="mytext2">Wait Key</p>
<p id="mytext3">Wait Key</p>
<script>
$(function(){
var str = "This run after DOM loaded";
$("#mytext1").html(str);
var KEY_UP = 38;
var KEY_DOWN = 40;
var KEY_LEFT = 37;
var KEY_RIGHT = 39;
//Set listener for keydown
$(document).keydown(function(e){
$("#mytext2").html("- keydown -");
switch(e.which){
case KEY_UP:
var top = parseInt($("#reddot").css("top")) - 10;
$("#reddot").css("top", top);
$("#mytext3").html("Key: UP / " + left + " : " + top);
break;
case KEY_DOWN:
var top = parseInt($("#reddot").css("top")) + 10;
$("#reddot").css("top", top);
$("#mytext3").html("Key: DOWN / " + left + " : " + top);
break;
case KEY_LEFT:
var left = parseInt($("#reddot").css("left")) - 10;
$("#reddot").css("left", left);
$("#mytext3").html("Key: LEFT / " + left + " : " + top);
break;
case KEY_RIGHT:
var left = parseInt($("#reddot").css("left")) + 10;
$("#reddot").css("left", left);
$("#mytext3").html("Key: RIGHT / " + left + " : " + top);
break;
}
});
//Set listener for keyup
$(document).keyup(function(e){
$("#mytext2").html("Wait Key");
$("#mytext3").html("Wait Key");
});
});
</script>
</body>
</html>
No comments:
Post a Comment